SQL DELETE Statement
Manipulating data is a fundamental aspect of database operations and in SQL, one of the most critical commands for this purpose is the SQL DELETE Statement. Today we will explore the syntax and application of the SQL DELETE Statement by providing examples and practical demonstrations.
You can start practicing the SQL DELETE Statement feature for free by creating a free account on FreeASPHosting.net and creating a free SQL Server Database.
KEY TAKEAWAYS:
- SQL DELETE Statement is used to remove records from a database table.
- To delete a specific row, you use the SQL DELETE FROM statement with a WHERE clause that specifies the condition for selecting the row to be removed.
- The power of the SQL DELETE Statement lies in its ability to delete multiple rows at once. By setting a condition that matches multiple records, you can delete them all in a single command.
- To delete all rows/records from a table in SQL, you can simply omit the WHERE clause in the DELETE statement.
- In situations where duplicate rows exist in a table, you can delete them by identifying the duplicates and deleting all but one occurrence. This is typically done using a subquery.
SQL DELETE Statement
SQL DELETE Statement is used to remove one or more records from a database table. The primary use case of this statement is when you need to erase certain data without impacting the overall structure of the table.
The basic syntax of a Delete Query in SQL is as follows:
DELETE FROM table_name WHERE condition;
In this syntax, "table_name" is the table you want to delete the data, and "condition" is a filter applied to select specific rows to be removed.
SQL Delete Row
The process of DELETE row from table SQL is a fundamental aspect of database operations. You would typically need to delete rows from a table when you want to eliminate specific records based on certain criteria.
Let's see an example to DELETE a row SQL. Suppose we have the following "Employees" table:
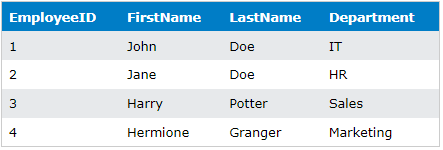
Now how to delete a row in SQL? Simple, to delete a record of an employee with an "EmployeeID" of 1 the SQL DELETE Statement would be:
DELETE FROM Employees WHERE EmployeeID = 1;
After executing this SQL DELETE ROW statement, the "Employees" table would look like this:
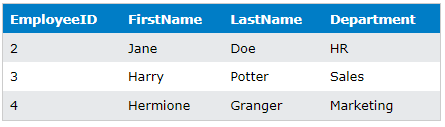
The row containing the information of “John Doe” from the “IT” department has been removed using SQL DELETE FROM!
SQL Delete Multiple Rows
The power of the SQL DELETE ROW statement comes from its flexibility. You can DELETE MULTIPLE ROWS IN SQL by setting a condition that matches multiple records. For example, if you need to remove all employees in the "Sales" department from the "Employees" table, the command would look like this:
DELETE FROM Employees WHERE Department = 'Sales';
After executing this DELETE FROM SQL statement, the "Employees" table would no longer include any employees from the "Sales" department. You can see how useful this SQL DELETE Rows feature is!
Delete All Rows From Table SQL
In SQL, you can DELETE ALL ROWS FROM TABLE SQL by not specifying a WHERE clause. The syntax is as follows:
DELETE FROM table_name;
For instance, to Delete All rows from our "Employees" table you will use the following DELETE data from table SQL statement:
DELETE FROM Employees;
After running this SQL DELETE Statement, the "Employees" table would be completely empty.
Caution: Exercise caution when deleting records from a table. Pay attention to the presence of the WHERE clause in the DELETE statement, as it defines which record(s) will be removed. If you overlook the WHERE clause, the deletion operation will delete all records in the table.
Delete Duplicate Rows in SQL
In some scenarios, you might encounter duplicate rows in your table, and it's essential to know how to DELETE DUPLICATE ROWS IN SQL. This process typically involves two steps: identifying duplicates and deleting them.
Assuming we have a "Duplicates" table with the following data:
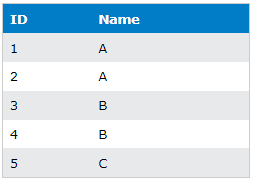
The duplicates in this table are rows with IDs 1 and 2, and 3 and 4. To delete these duplicates, you can use the following SQL DELETE Statement:
DELETE FROM Duplicates WHERE ID NOT IN (SELECT MIN(ID) FROM Duplicates GROUP BY Name);
After executing this SQL DELETE ROW statement, the "Duplicates" table would only include unique rows:
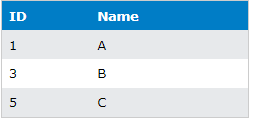
SQL DELETE IF EXISTS
In some scenarios, you might need to delete a row only if it exists in the table. You can achieve this using the SQL DELETE IF EXISTS approach. Although in SQL Server we can also use built-in IF EXISTS statement to check if a row exists and can perform further actions, in pure SQL this is typically done using a subquery. For instance, to delete an employee named "John Doe", only if his data exists in the "Employees" table, you would write:
DELETE FROM Employees WHERE EmployeeID = (SELECT EmployeeID FROM Employees WHERE FirstName = 'John' AND LastName = 'Doe');
If "John Doe" exists in the "Employees" table, this SQL DELETE Statement will remove his record.
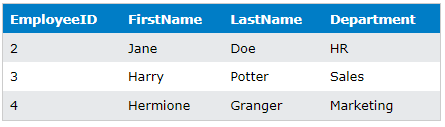
Delete Table SQL
In addition to deleting rows, if we want to know how to DELETE table in SQL entirely, then SQL also provided a way for that. It is performed using the DROP TABLE statement:
DROP TABLE table_name;
For instance, to delete our "Employees" table, we would use:
DROP TABLE Employees;
This SQL DELETE TABLE statement will remove the "Employees" table from the database entirely, including all its data and schema.
Delete From Join SQL
When working with relational databases, we often need to work with multiple tables simultaneously. It's important to know how to SQL Delete with Join. Let's consider two tables: "Employees" and "Departments".
DELETE e
FROM Employees e
JOIN Departments d ON e.DepartmentID = d.DepartmentID
WHERE d.DepartmentName = 'Sales';
Without table alias. The above delete SQL query will look like this.
DELETE Employees
FROM Employees
JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID
WHERE Departments.DepartmentName = 'Sales';
This SQL DELETE ROW FROM TABLE statement will remove all employees from the "Sales" department.
Final Words for SQL DELETE Statement
The SQL DELETE Statement is a powerful tool that allows us to manipulate our data effectively. However, it's crucial to use it cautiously. Given its potential to erase data permanently, it's advisable to always ensure you have a recent backup before running any DELETE row SQL statement. If you accidentally delete more records than intended, you may not be able to take back that unless you have a recent backup of your database.
Always double-check your conditions in the "WHERE" clause before executing the DELETE Statement SQL. Also, using transactions is a good practice as it let you roll back changes if something goes wrong.
Today you learned different ways you can use the DELETE SQL STATEMENT, from deleting single rows, multiple rows, and duplicate rows, to deleting entire tables. Armed with this knowledge, you can now comfortably manage and manipulate your database records.