CTE vs Temp Table
This article is designed to provide us a good understanding of three key components of SQL Server: Common Table Expression (CTE), Temporary Tables (Temp Tables), and Table Variables.
Through the course of the article, we will try to give you answer the most frequently asked questions related to these topics, backed up by practical SQL examples. This article is structured to help you learn about CTE in SQL Server, the creation of a Temp Table in SQL, and when to use Table Variables. The discussion will further extend to the comparison of CTE vs Temp Table, and the difference between them.
You can start practicing CTE, Temp Tables, and Table Variables for free by creating a free account on FreeASPHosting.net and creating a free SQL Server Database.
KEY TAKEAWAYS:
- Common Table Expressions (CTEs) are temporary in-memory result sets defined within the execution scope of a single statement. They used to break down a query into smaller, more manageable pieces, and they can also be used to reuse common sets of data.
- Temporary tables or Temp tables are used to store and process intermediate results. These tables are created and can be automatically deleted when they are no longer used.
- A SQL Local Temp table is only visible to the current session. It is deleted once the session that created it ends. A SQL Global Temp table is visible to all SQL Server connections, and it is deleted when the last connection which is referencing the table is closed.
- A SQL CTE is generated on the fly and not stored physically on the database (in memory), which makes it faster for small datasets. A Temp Table SQL is stored on tempdb, which makes it more efficient for larger datasets.
- Table Variables are a type of variable that allows you to store data rows. They behave similar to temp tables but have some differences.
Common Table Expression
A CTE or Common Table Expression is a temporary in-memory result set defined within the execution scope of a single SELECT, INSERT, UPDATE, DELETE, or CREATE VIEW statement. It is a more readable form of a subquery. They used to break down a query into smaller, more manageable pieces, and they can also be used to reuse common sets of data. Let's dive in further to understand how to create CTE in SQL, and when to use CTE in SQL.
Create CTE in SQL
Let's create a table first, to better understand how to write and run CTE SQL:
-- Create the table
CREATE TABLE SalesOrderHeader (
SalesOrderID INT,
SalesPersonID INT
);
-- Insert sample data
INSERT INTO SalesOrderHeader (SalesOrderID, SalesPersonID)
VALUES
(1, 101),
(2, 102),
(3, 101),
(4, 103),
(5, 102),
(6, 103),
(7, 101);
Now we are creating a SQL CTE using “SalesOrderHeader” table, and selecting data from CTE using the select query:
-- Create the CTE
WITH Sales_CTE (SalesPersonID, NumberOfOrders)
AS
(
SELECT SalesPersonID, COUNT(SalesOrderID)
FROM SalesOrderHeader
GROUP BY SalesPersonID
)
-- Query the CTE
SELECT SalesPersonID, NumberOfOrders
FROM Sales_CTE;
By running the above CTE SQL, we will get this Output:
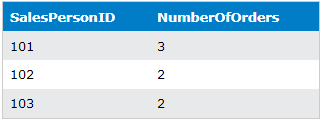
The "WITH" clause indicates the start of the CTE SQL. The query inside the parentheses runs first, and the results are stored in the CTE. The select query after the CTE then runs and uses the CTE SQL as if it were a standard table.
When to Use CTE in SQL?
You use a CTE in SQL when you want to create a temporary result set for one-time use. CTEs are great for recursive operations, and when you need to reference the same subquery multiple times within a query. CTEs can be used to simplify complex subqueries by breaking down into smaller, more manageable parts. It provides more readable and maintainable SQL code.
SQL Temp Table
Temporary tables or Temp tables are used to store and process intermediate results. These tables are created and can be automatically deleted when they are no longer used. Let's explore how to create temp table SQL, the difference between SQL local temp table and SQL global temp table, and when to use temp tables in SQL.
SQL Create Temp Table
CREATE TABLE #TempTable
(
UserID int,
UserName varchar(50),
UserEmail varchar(50)
);
INSERT INTO #TempTable
VALUES (1, 'User1', '[email protected]'), (2, 'User2', '[email protected]');
SELECT * FROM #TempTable;
In this SQL code, we first create a temporary table SQL "#TempTable" and then insert two rows of data. The result set from the "SELECT" statement would be:
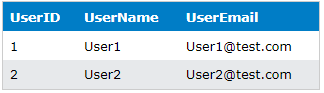
SQL Local Temp Table and SQL Global Temp Table
A SQL local temp table is only visible to the current session. It is deleted once the session that created it ends. A SQL global temp table is visible to all SQL Server connections, and it is deleted when the last connection which is referencing the table is closed. The local temp tables are defined with a single hashtag prefix ("#") while global temp tables are created with a double hashtag prefix ("##").
When to Use Temp Tables in SQL?
Temp tables are ideal when you are dealing with large amounts of data that need temporary storage for the duration of the current connection or session. It's also useful when complex calculations are performed, and there is a need for intermediate storage space.
CTE vs Temp Table
The main difference between CTE and Temp Table lies in their functionality and usage. A CTE SQL is best suited for recursive operations and subqueries used multiple times in a larger query, promoting readability and maintainability. On the other hand, Temp Tables are better for storing large amounts of data temporarily, particularly when performing complex calculations.
A SQL CTE is generated on the fly and not stored physically on the database (in memory), which makes it faster for small datasets. A Temp Table SQL is stored on tempdb, which makes it more efficient for larger datasets.
SQL Table Variable
A Table Variable is a type of variable that allows you to store data rows. They behave similar to temp tables, but have some differences.
Create Table Variable
Here's how to create a table variable:
DECLARE @EmployeeTable TABLE
(
EmployeeID int,
EmployeeName varchar(50),
Salary decimal
);
INSERT INTO @EmployeeTable
VALUES (1, 'John', 50000), (2, 'Jane', 60000);
SELECT * FROM @EmployeeTable;
The result set from the "SELECT" statement would be:
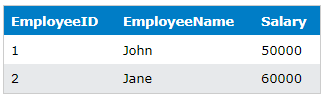
When to Use Table Variable in SQL?
Table Variables are useful when you have a smaller dataset, and you need a simple structure for storing the data temporarily. They provide a way to move rows between stored procedures and functions. They are also beneficial when you are performing a few queries, as Table Variables result in fewer recompilations of a query.
Final Words:
SQL CTE, Temp Tables, and Table Variables all have their specific use cases, and understanding them can help optimize your SQL queries. It is important to understand the CTE vs Temp Table comparison and when to use Table Variables to effectively make use of SQL Server's capabilities.