C# Switch Statement
The C# Switch statement is a versatile and dynamic programming construct that can significantly streamline code if used effectively.
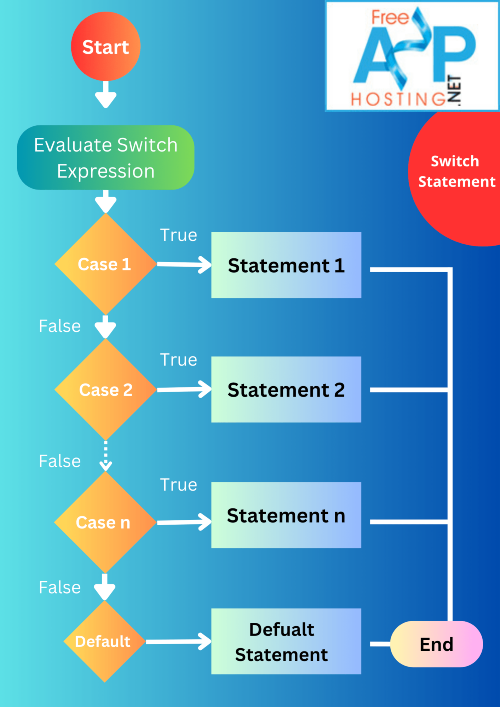
The functionality it offers is varied ranging from replacing multiple if-else statements to pattern matching which can greatly enhance code readability and maintainability. Today we will provide you with comprehensive understanding of the C# Switch Statement providing clear examples for many of its applications.
You can create a free account on FreeASPHosting.net, host your ASP.NET application, can create a free SQL Server Database and start practicing C# Swtich Statement and other programming constructs!
KEY TAKEAWAYS:
- The C# Switch Statement can replace multiple if-else statements and offer functionalities such as pattern matching, enhancing code readability and maintainability.
- Basic Syntax: The C# Switch Statement allows a variable to be tested for equality for a list of values (cases). Each case represents a possible value, and the corresponding code block is executed if a match is found.
- Break and Default: The break keyword is used to terminate the switch case once a match is found, preventing the execution of other cases. The default keyword provides a pathway for the program to follow if none of the cases match the switch expression.
- Return and Goto: The return keyword can be used in a C# Switch case to return a value from a method once a match is found. The goto keyword allows jumping from one case to another, providing greater control over the flow of execution.
- Switch vs If-Else: The choice between a switch statement and an if-else structure depends on the nature of the condition. Switch statements are more readable and efficient when testing a single variable against a series of exact equality conditions, while if-else structures may be more appropriate for complex conditions or multiple variable tests.
C# Switch Statement
Let's start with the basics. The C# Switch case statement is a control flow statement that allows the variable to be tested for equality (also can use relational operators) for a list of values. Each value is a case, and the variable is checked for each case.
Here's a simple example of the C# Switch Statement:
int number = 3;
switch (number)
{
case 1:
Console.WriteLine("One");
break;
case 2:
Console.WriteLine("Two");
break;
case 3:
Console.WriteLine("Three");
break;
default:
Console.WriteLine("No match");
break;
}
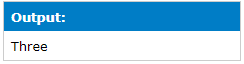
In the above code, the "number" variable is evaluated in the switch statement C#, and each case is tested for a match. The keyword break is used after each case. If "number" is not matched with any case, it executes the code block following the default keyword.
C# Switch Case
The fundamental element of a C# Switch Statement is the case. Each case in a C# Switch Statement represents a possible value that the switch expression can match.
A Switch Case C# contains a value that the switch expression is compared against. If there's a match, the corresponding block of code is executed.
A C# case must always be accompanied by the keyword break, which is used to exit the switch block once a match is found, preventing the execution of other cases.
C# Switch Break
The break keyword in a C# Switch Statement is used to terminate the switch case once a match is found. Once a case matches the switch expression, the corresponding block of code is executed, and the break keyword takes the control out of the switch block.
If the break keyword is omitted after a case, the code execution would continue to the next case, causing unintended behavior. This is often referred to as "fall-through" and is not generally allowed in Switch C# statements.
C# Switch Default
The default keyword in a C# Switch Statement provides a pathway for the program to follow if none of the cases match switch expression. Essentially, it's the "else" part of the C# Switch Statement.
C# Switch Return
Return keyword can be used in a C# Switch case to return a value from a method once a match is found. This can be especially useful when using methods that need to return a value based on a condition.
Here's a C# Switch Statement example with Return:
public string NumberToString(int number)
{
switch (number)
{
case 1:
return "One";
case 2:
return "Two";
case 3:
return "Three";
default:
return "No match";
}
}
In this example, the method "NumberToString" returns a string based on the input number. The C# Switch return is used to return the corresponding string.
C# Goto
The Goto keyword can be used within a Switch C# Statement to jump from one case to another. This allows for greater control over the flow of execution within the C# Switch case.
Look at this C# Goto example:
int number = 2;
switch (number)
{
case 1:
Console.WriteLine("One");
break;
case 2:
Console.WriteLine("Two");
goto case 1;
case 3:
Console.WriteLine("Three");
break;
default:
Console.WriteLine("No match");
break;
}
In this Switch Case C# example, if the "number" variable is 2, it prints "Two" and then jumps to case 1, printing "One".
Switch Statements Grouped Cases in C#
In C#, multiple values can be tested in a single case by separating them with a comma. This allows you to group cases that should have the same result.
Look at the following C# Switch Statement example:
int number = 2;
switch (number)
{
case 1:
case 2:
case 3:
Console.WriteLine("Number is between 1 and 3");
break;
default:
Console.WriteLine("Number is not between 1 and 3");
break;
}
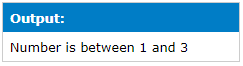
In this C# Switch case example, if "number" is 1, 2, or 3, the same block of code is executed. This is a way to group multiple cases together.
C# Nested Switch
A nested switch is a switch statement inside another switch statement. This allows for more complex logic to be implemented within a C# Switch case.
C# Nested Switch Example:
int outerNumber = 1;
int innerNumber = 2;
switch (outerNumber)
{
case 1:
switch (innerNumber)
{
case 1:
Console.WriteLine("One-One");
break;
case 2:
Console.WriteLine("One-Two");
break;
default:
Console.WriteLine("One-No match");
break;
}
break;
case 2:
Console.WriteLine("Two");
break;
default:
Console.WriteLine("No match");
break;
}
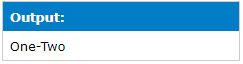
In this Switch Case C# example, we have an outer switch and an inner switch. The inner switch is executed if the outerNumber is 1.
C# Switch vs If Else
The choice between switch statement c# and an if-else structure often depends on the nature of the condition to be tested. C# Switch Statements are typically more readable and efficient when testing a single variable against a series of exact equality conditions. However, for more complex conditions, such as ranges or multiple variable tests, an if-else structure might be more appropriate.
For example, the following switch statement:
switch (number)
{
case 1:
Console.WriteLine("One");
break;
case 2:
Console.WriteLine("Two");
break;
default:
Console.WriteLine("No match");
break;
}
Would be more verbose and less clear if written with if-else:
if (number == 1)
{
Console.WriteLine("One");
}
else if (number == 2)
{
Console.WriteLine("Two");
}
else
{
Console.WriteLine("No match");
}
C# Switch with Enum
The C# Switch Statement can also work with enum values, allowing for more readable code when working with enumerated types.
C# Switch with Enum example:
enum Days { Monday, Tuesday, Wednesday }
Days day = Days.Tuesday;
switch (day)
{
case Days.Monday:
Console.WriteLine("It's Monday");
break;
case Days.Tuesday:
Console.WriteLine("It's Tuesday");
break;
case Days.Wednesday:
Console.WriteLine("It's Wednesday");
break;
default:
Console.WriteLine("No match");
break;
}
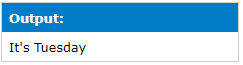
In this C# Switch case example, the "day" variable is of an enumerated type. The C# Switch statement matches the cases with enum values.
C# Switch with Relational Operators
A C# Switch Statement with relational operators allows us to do different actions based on the comparison of a variable or expression with multiple conditions using relational operators such as greater than, less than, equal to, etc.
Here's an example of a C# Switch Statement using relational operators:
int num = 10;
switch (num)
{
case < 0:
Console.WriteLine("The number is negative");
break;
case > 0:
Console.WriteLine("The number is positive");
break;
case 0:
Console.WriteLine("The number is zero");
break;
default:
Console.WriteLine("Invalid number");
break;
}
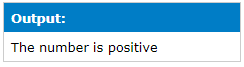
C# Switch Pattern Matching
With the introduction of C# 7.0, C# Switch Statements got a lot more powerful with the addition of pattern matching capabilities. This allows a switch statement to not only check values but also to decompose data types in a manner similar to destructuring.
For instance:
object number = 1;
switch (number)
{
case int i:
Console.WriteLine($"It's an integer: {i}");
break;
case string s:
Console.WriteLine($"It's a string: {s}");
break;
default:
Console.WriteLine("It's something else");
break;
}
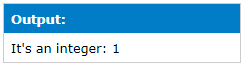
In this C# Switch Pattern Matching example, the "number" variable is an object. Depending on its actual type at runtime, a different case will be matched.
Important Points to Remember for C# Switch Statement
- Each C# Case must be followed by a break, return, or goto keyword to terminate the execution of that case.
- The default case in a C# Switch Statement is optional, and it's used when none of the cases match the switch expression.
- Pattern matching in C# Switch Statements allows for more complex case conditions, including type checking and data decomposition.
- C# Switch Statements work with primitive data types, enums, strings, or special types that have the ability to compare equality.
- In general, C# Switch Statements are more efficient than if-else chains when testing a variable against a series of constant values.
Final Words for C# Switch Statement
C# Switch Statements are a valuable tool for developers. They allow for clean, easy-to-read code that can handle complex conditionals. With the addition of pattern-matching capabilities in recent C# updates, C# Switch has become even more powerful and versatile. Understanding how to use it effectively can greatly improve the quality of your code.
Let’s go ahead and read our other advanced C# articles on Reflection in C#, Abstract Class vs Interface C#, and C# Lambda function to learn more.