Forms in practice
Web form development consists of placing controls onto a UI.
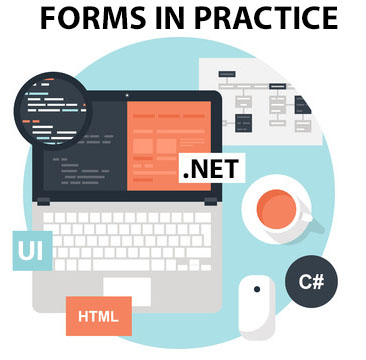
The controls are then analyzed to decide on appropriate properties, events, and methods. Interfaces utilize two types of controls: HTML controls and Web Form controls.
HTML CONTROLS
These controls resemble and behave like standard HTML elements. Simply select a control from the HTML Toolbox tab, and draw the control in the place desired. Any existing HTML element can be marked to run as a control by adding "runat=server" to the element's tag, or by right-clicking the element in Visual Studio and selecting “Run as Server Control.”
A few of the available controls and their code are presented below for review:
HTML Control | Action | Code |
---|---|---|
Button | It produces a button for click events. | <input type=button runat=server> |
Text Field | It provides a small text input area. | <input type=text runat=server> |
File Field | It provides a text field and a Browse button for file selection. | <input type=file runat=server> |
Dropdown | It provides a list of options with only a single option selected at once. | <select><option></opti on></select> |
Radio Button | It provides a list of options with only a single option selected at once, and all options in view. | <input type=radio runat=server> |
CheckBox | It provides checkboxes for selecting options. | <input type=checkbox runat=server> |
Text Area | It provides a large text input area. | <input type=textarea runat=server> |
Submit Button | It provides a POST of the form data on click. | <input type=submit runat=server> |
All controls produce standard HTML. ID attributes can also be assigned for client-side code.
WEB FORM CONTROLS
The creation and function of Web Form controls works the same way as HTML. They perform an action, and render HTML (e.g., a DropDownList binds to a data source and then outputs <select> and <option>). Controls do not map to a particular markup. The control renders appropriate markup such as WML on a cell phone.
Web Form controls inherit from the System.Web.UI.WebControls class. The class implements a set of properties all controls possess. Review some of these properties below:
- Enabled
- TabIndex
- Font
- Width
- Visible
The .NET framework supplies a number of different types of controls, and some nearly correspond 1- to-1 with HTML. Some offer additional information when posted to the server, and others allow the display of data in tabular or list format. Review a list of some of these Web Form server-side controls and events below:
Control | Description | Event | Code |
---|---|---|---|
TextBox | It provides an input area. | TextChanged | <asp:TextBox id=TextBox1 runat="server"></asp:Text Box> |
RadioButton | It displays a button for selection. | CheckChanged | <asp:RadioButton id=RadioButton1 runat="server"></asp:Radi oButton> |
ListBox | It displays a List box. | SelectedIndexChange d | <asp:ListBox id=ListBox1 runat="server"> |
Calendar | It displays an HTML calendar. | SelectionChanged, VisibleMonthChanged, DayRender | <asp:Calendar id=Calendar1 runat="server"></asp:Cale ndar> |
DropDownList | It displays a dropdown list. | SelectIndexChanged | <asp:DropDownList id=DropDownList1 runat="server"></asp:Drop DownList> |
The browser detected by the application changes the output of all controls with the goal of optimizing appearance and performance.
FIELD VALIDATOR CONTROLS
This type of control validates data on the client without a “roundtrip.” Typical function sends data provided by the user back to the server for validation, but through ASP.NET, the control performs this function. The control creates client-side JavaScript code for this purpose. Review code for CompareValidator below:
<asp:CompareValidator id=CompareValidator1 runat="server" ErrorMessage= "CompareValidator"> </asp:CompareValidator>
It allows the contents of a control to be compared to the contents of another to determine if the contents match. If no match is found, an error message set by the developer appears.
ASP.NET also allows the creation of custom controls.
FORM FUNCTION
Events fire in a particular order on the initialization and loading of a Web Form, and other events fire in response to user action. The sequence of a Web Form begins with loading, advances to rendering, and ends with unloading events. In this process, different class procedures execute, and a DLL encapsulates requested pages, tags, and code before any processing occurs.
CREATE A FORM
Creating a form only requires adding (drag and drop) elements to a physical page to satisfy UI requirements, and then simply setting properties based on the desired function. Edit code for each control by double-clicking the control. A window appears with its associated code (i.e., the automatically generated code based on settings).