LINQ GroupBy
If you want to learn about data grouping using LINQ GroupBy, you're in the right place! LINQ Grouping is a potent tool that makes querying data efficient.
LINQ has become a game-changer in the realm of C# programming, allowing us to easily query and manipulate data. And among the many LINQ methods, there's one special we'll be exploring today: the LINQ GroupBy method. So, buckle up and get yourself ready to learn data grouping using Groupby LINQ C#.
Today, our focus will be LINQ Group By. When data seems difficult to organize and analyze, GroupBy LINQ is your best friend. LINQ GroupBy neatly organizes data into groups based on a given key.
KEY TAKEAWAYS:
- LINQ GroupBy is a powerful tool for efficiently organizing and analyzing data in C#.
- LINQ GroupBy can be used with both Query syntax and Method syntax. Query syntax offers a declarative SQL like way to express data queries, while Method syntax provides a more concise and functional way to write queries.
- By using LINQ GroupBy, you can easily group data objects based on a single property or multiple properties, enabling you to organize and categorize data effectively.
- LINQ GroupBy also allows you to perform additional operations on the groups created, such as aggregating data, applying transformations, or selecting specific properties from each group.
- It is important to consider performance when using LINQ GroupBy with large datasets. Optimizing queries, choosing efficient data structures, and leveraging indexing can help improve performance. Additionally, testing and debugging queries are essential to ensure accurate results.
LINQ GroupBy
LINQ Group By is a versatile tool. You are going to explore some of its usage in C#, using both Query syntax and Method syntax. Don’t worry you will learn the difference in the next section. Let's dive headfirst into the LINQ GroupBy C#. The basic usage of C# Group By is very smooth. Here's how it works:
//Define a list of fruits
List<string> fruits = new List<string>
{
"apple", "banana", "orange", "apple", "grape", "banana", "apple"
};
// Group the fruits using Query syntax
var fruitsGrouped = from fruit in fruits
group fruit by fruit;
// Group the fruits using method syntax
var fruitsGrouped = fruits.GroupBy(fruit => fruit);
You've just grouped fruits by their type using LINQ Group By. This will yield groups of apples, bananas, oranges, and grapes. Here is a full C# console working code for the above. You can uncomment the Method syntax part to test that, but both will return the same results.
using System;
using System.Collections.Generic;
using System.Linq;
public class Program
{
public static void Main(string[] args)
{
// Define a list of fruits
List<string> fruits = new List<string>
{
"apple", "banana", "orange", "apple", "grape", "banana", "apple"
};
// Group the fruits using Query syntax
var fruitsGrouped = from fruit in fruits
group fruit by fruit;
// Group the fruits using method syntax
// var fruitsGrouped = fruits.GroupBy(fruit => fruit);
// Print the grouped fruits
foreach (var group in fruitsGrouped)
{
Console.WriteLine($"Fruit: {group.Key}, Count: {group.Count()}");
}
}
}
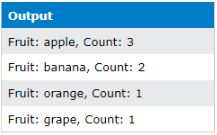
LINQ GroupBy - Query Syntax Vs Method Syntax
Let’s first see the difference between these two types of the syntax used in LINQ queries, if you are not already aware, as we are going to use both in our LINQ GroupBy C# examples.
Query Syntax:
Query Syntax in LINQ offers a declarative and intuitive way to express your data queries. When it comes to grouping data in C#, the Group in LINQ is the first approach used by many developers. Let's have a look at query syntax for the C# LINQ Group By. But don’t bother if it seems difficult, easy examples with explanations are ahead. This is just to give you how-know of the LINQ Group By query syntax:
var groupedData =
from item in collection
group item by item.Property into grouped
select grouped;
Here, we have “collection”, representing the data source, and “item”, representing an individual element in the collection. With the power of the LINQ Grouping feature, we can group items based on their Property by using LINQ Group By. The result will be a sequence of grouped elements. You will also learn what this LINQ Group By Into, in the upcoming section.
Method Syntax:
For functional style and short syntax coding, method syntax is ideal in C# LINQ Group, giving you a more concise and composable way to write queries. To wield the power of LINQ GroupBy with method syntax, check out the following example:
var groupedData = collection.GroupBy(item => item.Property);
We're still using “collection” as our data source, but this time. Using the C# GroupBy method, we group items based on their Property.
Linq Group By Into
LINQ Group Into is where we start getting deep into the data. The Group into clause in LINQ Group enables you to modify or analyze the groups created by the LINQ Group By clause. It provides the ability to perform extra operations on the groups, such as combining data, applying transformations, or selecting specific properties from each group.
We can group into a new type that contains the key and the number of occurrences. Check it out:
// Group the fruits and project into a new anonymous type using Query syntax
var fruitsGrouped = from fruit in fruits
group fruit by fruit into fruitGroup
select new { FruitType = fruitGroup.Key, FruitCount = fruitGroup.Count() };
// Group the fruits and project into a new anonymous type using method syntax
var fruitsGrouped = fruits.GroupBy(fruit => fruit)
.Select(fruitGroup => new { FruitType = fruitGroup.Key, FruitCount = fruitGroup.Count() });
// Print the grouped fruits
foreach (var group in fruitsGrouped)
{
Console.WriteLine($"Fruit: {group.FruitType}");
Console.WriteLine("Occurrences: " + group.FruitCount);
Console.WriteLine();
}
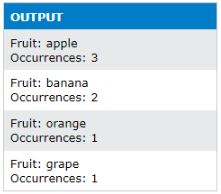
We added the “into” keyword after the group by clause, which allows us to assign a new range variable (fruitGroup) to represent each group. Then, we use the select clause to create a new anonymous type with properties FruitType (group key) and FruitCount (count of items in the group).
Linq Each
The LINQ ForEach() method allows us to execute an action on each element of a sequence. The syntax for the ForEach() method is as follows:
var numbers = new List<int> { 1, 2, 3, 4, 5 };
numbers.ForEach(x => Console.WriteLine(x));
/* This code will print the following output to the console:
1
2
3
4
5
*/
With LINQ GroupBy, for any products collection, we can use like this:
var groupedProducts = products.GroupBy(p => p.Category);
groupedProducts.ToList().ForEach(group =>
{
Console.WriteLine($"Category: {group.Key}");
group.ToList().ForEach(product => Console.WriteLine($" Product: {product.Name}, Price: {product.Price}"));
});
LINQ GroupBy - Some more Examples
Let’s have some more examples with LINQ GroupBy. You have to create the required classes to run this code.
LINQ GroupBy - Grouping Objects by a Single Property:
If you have bunch of students, and you want to group them based on their grades. You can use LINQ Group By effectively in this scenario. We already covered an example similar to this, just another for exercise:
var students = new List<Student>
{
new Student { Name = "John", Age = 20, Grade = "A" },
new Student { Name = "Jane", Age = 21, Grade = "B" },
new Student { Name = "Alex", Age = 20, Grade = "A" },
new Student { Name = "Emily", Age = 21, Grade = "C" }
};
// Group the students by grade using query syntax
var groupedStudents =
from student in students
group student by student.Grade into grouped
select grouped;
// Group the students by grade using method syntax
var groupedStudents = students.GroupBy(student => student.Grade);
foreach (var group in groupedStudents)
{
Console.WriteLine($"Students with grade {group.Key}:");
foreach (var student in group)
{
Console.WriteLine(student.Name);
}
Console.WriteLine();
}
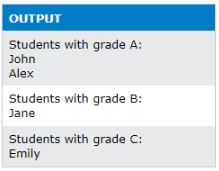
Here we grouped the students by their grades using the LINQ Group By clause. Now we can see each group of students with their respective grades.
LINQ GroupBy - Grouping Objects by Multiple Properties:
Next we have a more challenging task using C# LINQ GroupBy: grouping objects by multiple properties. If the employees are grouped not only by their departments but also by their ages.
Here’s an example:
var employees = new List<Employee>
{
new Employee { Name = "John", Department = "HR", Age = 30 },
new Employee { Name = "Jane", Department = "Engineering", Age = 35 },
new Employee { Name = "Alex", Department = "HR", Age = 30 },
new Employee { Name = "Emily", Department = "Sales", Age = 25 }
};
// Group the employees by department and age using method syntax
var groupedEmployees = employees.GroupBy(employee => new { employee.Department, employee.Age });
foreach (var group in groupedEmployees)
{
Console.WriteLine($"Employees in {group.Key.Department} department, age {group.Key.Age}:");
foreach (var employee in group)
{
Console.WriteLine(employee.Name);
}
Console.WriteLine();
}
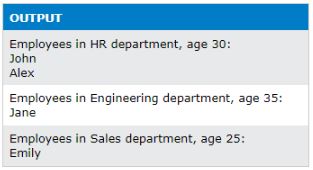
By crafting a powerful anonymous object, we can now group employees based on their departments and ages simultaneously using C# LINQ GroupBy.
LINQ GroupBy - Grouping and Aggregating Data
Now we will do Grouping and aggregating data using Group in LINQ for a collection of sales transactions. We want to Group in LINQ by year while calculating the total sales for each year, and also sort them by date.
Here is a complete console code:
// Import the necessary libraries
using System;
using System.Collections.Generic;
using System.Linq;
// Create a new Transaction class with two properties
public class Transaction
{
// Property to hold the amount of the transaction
public decimal Amount { get; set; }
// Property to hold the date of the transaction
public DateTime Date { get; set; }
}
public class Program
{
// Main method
public static void Main()
{
// Create a list of transactions
var transactions = new List<Transaction>
{
// Initialize transactions with amount and date
new Transaction { Amount = 100, Date = new DateTime(2022, 1, 1) },
new Transaction { Amount = 200, Date = new DateTime(2022, 2, 1) },
new Transaction { Amount = 150, Date = new DateTime(2023, 1, 1) },
new Transaction { Amount = 300, Date = new DateTime(2023, 2, 1) }
};
// Sort the transactions by date and then group them by year
var groupedTransactions = transactions
.OrderBy(transaction => transaction.Date) // Order transactions by date
.GroupBy(transaction => transaction.Date.Year); // Group transactions by year
// Loop over each group of transactions
foreach (var group in groupedTransactions)
{
// Calculate the total sales for each year
decimal totalSales = group.Sum(transaction => transaction.Amount);
// Print the year and the total sales for that year
Console.WriteLine($"Year: {group.Key}, Total Sales: {totalSales}");
}
}
}
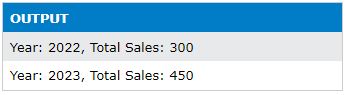
By sorting and grouping the transactions by the date and year respectively, using groupby and orderby, and using the Sum method, we can calculate the total sales for each year sorted by date. GroupBy OrderBy is widely used together.
Group By LINQ - Understanding The Power Of GroupBy LINQ C#
So, what are the benefits of GroupBy LINQ C#? It saves time, reduces complexity, and, quite simply, makes your life easier.
The importance of LINQ Grouping lies in its ability to reshape raw data into meaningful insights.
LINQ GroupBy C# - Common Pitfalls and Best Practices
Here's how you can troubleshoot your LINQ GroupBy C#. Always remember to check your keys and make sure they're not null. Also, be cautious about deferred execution. LINQ GroupBy is a bit of a procrastinator and doesn't execute until absolutely necessary (you can use ToLookup for immediate execution).
And a word of caution: don't confuse Link Group By with LINQ Group By. The Link is the misspelled word for LINQ.
LINQ Grouping - Tips and Tricks
For LINQ Grouping, here we have tips and tricks to make the most of the C# LINQ GroupBy method:
- Choose meaningful property names for LINQ Grouping: Give your properties expressive names. It makes the resulting groups more meaningful and easier to grasp.
- Anonymous objects for complex LINQ Grouping: When things get tricky with C# LINQ GroupBy multiple properties, create anonymous objects to simplify your groupings.
- Embrace aggregation functions: The C# LINQ GroupBy method pairs beautifully with aggregation functions like LINQ Sum, LINQ Count, and LINQ Average. You can derive meaningful insights and metrics from your grouped data.
- Performance: LINQ Grouping large datasets can slow things down. Optimize your queries, choose efficient data structures, and consider leveraging indexing for a performance boost.
- Test and debug: Complex LINQ Grouping can be challenging. Don't forget to test and debug your queries to ensure they work perfectly. Inspect the intermediate results and validate your code.
Final Words
We have learned a lot about LINQ Grouping using the C# LINQ GroupBy method. We have seen the power of both query syntax and method syntax and have practiced through multiple examples.
So go forth and read our other detailed articles on LINQ like LINQ Expressions, C# LINQ Lambda, and C# Lambda in List, and cast your C# LINQ GroupBy queries with confidence. So, get out there and start Grouping!