C# String Split
Download Sample Source CodeManipulating strings, such as knowing how to split a string C#, is an essential skill for any C# programmer. It allows us to divide a given string into multiple substrings based on a specified delimiter.
This functionality is crucial in scenarios where we need to extract meaningful data from a larger string or parse text-based inputs. The String.Split method in C# provides a convenient way to accomplish this task efficiently and effectively.
Although we will cover how to use C# Split() method with different examples, to give you a deep understanding of its syntax and usage, at the end of this comprehensive article, we will show you most of the C# Split() method overloads in a table with their description, and code examples for each, how they can help you to Split The String In C# in multiple ways!
KEY TAKEAWAYS:
- String splitting is an essential skill for C# programmers, allowing them to divide a given string into multiple substrings based on a specified delimiter.
- The primary method for string splitting in C# is the Split() method, which can split a string based on specified conditions and return an array of substrings.
- The C# Split() method can be used with different delimiters for C# String Split, including single characters, multiple characters, multiple delimiters, or even regular expressions.
- The C# Split() method provides flexibility through its various overloads, allowing you to control the number of substrings returned, remove empty substrings, and specify multiple delimiters.
- Understanding and effectively using the C# String Split method is crucial for tasks such as processing user input, parsing data, and manipulating strings, and it can significantly enhance your programming capabilities.
C# String Split
Split the String in C#, is to break it up into several substrings based on a specified delimiter. In other words, string splitting is the process of breaking up a string into multiple parts, based on a certain criterion. This function is incredibly useful when processing user input, parsing data, or just generally working with strings.
The primary method to split the string in C# is the Split() method. This is a built-in method that allows you to split a string C# based on specified conditions, returning an array of substrings.
Split() Syntax
In its simplest form, the C# Split() method can be called without any parameters, which splits the string on white spaces:
string sentence = "This is a test sentence.";
string[] words = sentence.Split();
foreach (string word in words)
{
Console.WriteLine(word);
}
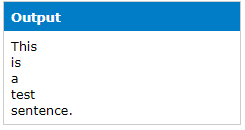
It can also take one or more characters as delimiters and returns an array of substrings. Here's the basic syntax:
string[] substrings = inputString.Split(delimiters);
We can split string in C# by specifying a single character delimiter. For example, let's C# string split a sentence into words using an underscore ('_') as the delimiter:
string sentence = "Hello_how_are_you_today?";
string[] words = sentence.Split('_');
foreach (string word in words)
{
Console.WriteLine(word);
}
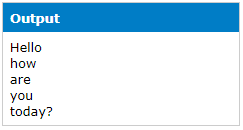
C# String Split Example
C# Split String By Comma
The String.Split C# method is very flexible and can use different characters or strings as delimiters. Here's an example of using a comma as a delimiter:
string csv = "one,two,three";
string[] items = csv.Split(',');
foreach(string item in items)
{
Console.WriteLine(item);
}
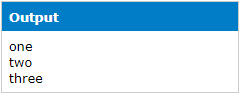
C# Split String By Multiple Characters
The C# Split() method can take an array of characters to use as delimiters. Let's split a string using a comma ( , ) a space ( ' ' ), and semicolon ( ; ) as delimiters:
string input = "one,two three;four";
char[] delimiters = {',', ' ', ';'};
string[] items = input.Split(delimiters);
foreach(string item in items)
{
Console.WriteLine(item);
}
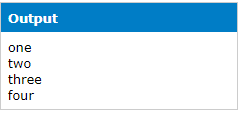
C# Split String By Multiple Delimiters
Apart from single characters, you can split a string using a delimiter string or an array of strings. This is useful when you have multiple possible delimiters. Look at this example!
string sentence = "I like apples, bananas, and oranges";
string[] fruits = sentence.Split(new[] { ", ", " and " }, StringSplitOptions.None);
foreach (string fruit in fruits)
{
Console.WriteLine(fruit);
}
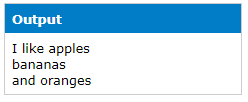
C# String Split - Return a Specific Number of Substrings
The C# String Split method allows you to control the number of substrings returned by specifying the maximum number of substrings desired. The C# Split() method can be given a count, which limits the number of substrings returned. Consider the following example:
string input = "one two three four five";
string[] firstTwo = input.Split(' ', 3);
foreach(string item in firstTwo)
{
Console.WriteLine(item);
}
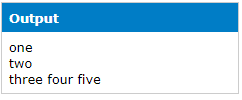
Look at another one:
string sentence = "The quick brown fox jumps over the lazy dog";
string[] words = sentence.Split(' ', 3);
foreach (string word in words)
{
Console.WriteLine(word);
}
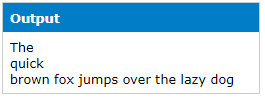
C# Regex Split
If you need more advanced splitting capabilities, you can utilize regular expressions with the C# Regex Split method. This allows you to split a string based on complex patterns. Here's an example:
string input = "one123two456three";
string[] items = System.Text.RegularExpressions.Regex.Split(input, @"\d+");
foreach(string item in items)
{
Console.WriteLine(item);
}
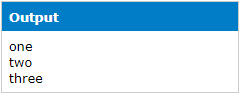
C# Join Strings
After splitting a string, you may want to rejoin the substrings in a different order or with a different delimiter. This can be done using the string.join c# method. You also have to include using System.Linq namespace.
string sentence = "The quick brown fox";
string[] words = sentence.Split(' ');
string reversed = string.Join(" ", words.Reverse());
Console.WriteLine(reversed);
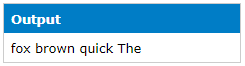
C# String Split - Method Overloads
There are several overloads of the Split() method to C# String Split, which give you more options for the C# split string. These overloads allow you to specify multiple delimiters, control whether empty substrings are included, and limit the number of substrings returned. We will cover 6 here!
The count parameter in some overloads of C# string split limits the maximum number of substrings that will be returned.
The StringSplitOptions enum determines such as whether empty substrings should be included or removed from the resulting array or trim whitespace from substrings..
Method | Description |
---|---|
Split(char[] separator) | Splits string into substrings that are based on the characters in an array. |
Split(char[] separator, int count) | Splits string into a maximum number of substrings that are based on the characters instead of splitting it at every point where the separator occurs. |
Split(char[] separator, StringSplitOptions options) | Splits string into substrings that are based on the characters in an array and options, like removing empty substrings from the resulting array if specified by the options parameter. |
Split(char[] separator, int count, StringSplitOptions options) | Splits string into a maximum number of substrings based on the characters, and options like removing empty substrings from the resulting array if specified by the options parameter. |
Split(string[] separator, StringSplitOptions options) | Splits string into substrings that are based on the strings in an array and options parameter. |
Split(string[] separator, int count, StringSplitOptions options) | Splits string into a maximum number of substrings based on the strings in an array and options parameter. |
You can see the implementation of each C# string split overload mentioned above, in the following detailed console example.
using System;
class Program
{
static void Main()
{
string str = "one,two,,three,four";
// Method: Split(params char[] separator)
// Description: Splits a string into substrings that are based on the characters in an array.
var result = str.Split(',');
foreach (var item in result)
Console.WriteLine(item);
// Output:
// one
// two
// (empty string)
// three
// four
Console.WriteLine();
// Method: Split(char[] separator, int count)
// Description: Splits a string into a maximum number of substrings that are based on the characters instead of splitting it at every point where the separator occurs.
result = str.Split(',', 3);
foreach (var item in result)
Console.WriteLine(item);
// Output:
// one
// two
// ,three,four
Console.WriteLine();
// Method: Split(char[] separator, StringSplitOptions options)
// Description: Splits a string into substrings that are based on the characters in an array and options, like removing empty substrings from the resulting array if specified by the options parameter.
result = str.Split(new char[] { ',' }, StringSplitOptions.RemoveEmptyEntries);
foreach (var item in result)
Console.WriteLine(item);
// Output:
// one
// two
// three
// four
Console.WriteLine();
// Method: Split(char[] separator, int count, StringSplitOptions options)
// Description: Splits a string into a maximum number of substrings based on the characters, and options like removing empty substrings from the resulting array if specified by the options parameter.
result = str.Split(new char[] { ',' }, 3, StringSplitOptions.RemoveEmptyEntries);
foreach (var item in result)
Console.WriteLine(item);
// Output:
// one
// two
// three,four
Console.WriteLine();
// Method: Split(string[] separator, StringSplitOptions options)
// Description: Splits a string into substrings that are based on the strings in an array and options parameter.
result = str.Split(new string[] { ",," }, StringSplitOptions.RemoveEmptyEntries);
foreach (var item in result)
Console.WriteLine(item);
// Output:
// one,two
// three,four
Console.WriteLine();
// Method: Split(string[] separator, int count, StringSplitOptions options)
// Description: Splits string into maximum number of substrings based on the strings in an array and options parameter.
result = str.Split(new string[] { "," }, 3, StringSplitOptions.RemoveEmptyEntries);
foreach (var item in result)
Console.WriteLine(item);
// Output:
// one
// two
// three,four
}
}
C# String Split Performance
The performance of C# string split is generally quite good, but it can be impacted by several reasons such as the size of the string, the complexity of the delimiter, and the options used. In general, simple splits are faster than using regular expressions or complex delimiters.
Recap of C# String Split
Throughout this article, we've seen how to split a string C# in a variety of ways using the C# Split() method and its various overloads.
Understanding how to C# Split String effectively is an important skill in C#. Whether you're parsing user input, processing text files, or just generally manipulating strings, the ability to split the string in C# will come in handy in many situations. As always, practice is key to mastering these concepts, so be sure to experiment with the code examples and explore the C# Split() method in more depth on your own.